- Mesaj
- 810
- Çözümler
- 28
- Beğeni
- 746
- Puan
- 839
- Ticaret Puanı
- 0
Mob Target sisteminde normal paylaşılanda bu kadar detay yoktur eklemek isteyenler olursa module'leri paylaşıyorum.
PythonNonPlayer.h
PythonNonPlayer.cpp
PythonNonPlayerModule.cpp
" ? " Tuşuna basınca açılacak yer;
uitarget.py
Source :
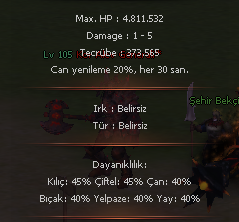
PythonNonPlayer.h
C:
#ifdef ENABLE_TARGET_INFORMATION_SYSTEM
// TARGET_INFO
DWORD GetMonsterMaxHP(DWORD dwVnum);
DWORD GetMonsterRaceFlag(DWORD dwVnum);
DWORD GetMonsterLevel(DWORD dwVnum);
DWORD GetMonsterDamage1(DWORD dwVnum);
DWORD GetMonsterDamage2(DWORD dwVnum);
DWORD GetMonsterExp(DWORD dwVnum);
float GetMonsterDamageMultiply(DWORD dwVnum);
DWORD GetMonsterST(DWORD dwVnum);
DWORD GetMonsterDX(DWORD dwVnum);
bool IsMonsterStone(DWORD dwVnum);
DWORD GetMobResist(DWORD dwVnum, BYTE bResistNum);
BYTE GetMobRegenCycle(DWORD dwVnum);
BYTE GetMobRegenPercent(DWORD dwVnum);
DWORD GetMobGoldMin(DWORD dwVnum);
DWORD GetMobGoldMax(DWORD dwVnum);
#endif
PythonNonPlayer.cpp
C:
#ifdef ENABLE_TARGET_INFORMATION_SYSTEM
DWORD CPythonNonPlayer::GetMonsterMaxHP(DWORD dwVnum)
{
const CPythonNonPlayer::TMobTable * c_pTable = GetTable(dwVnum);
if (!c_pTable)
{
DWORD dwMaxHP = 0;
return dwMaxHP;
}
return c_pTable->dwMaxHP;
}
DWORD CPythonNonPlayer::GetMonsterRaceFlag(DWORD dwVnum)
{
const CPythonNonPlayer::TMobTable * c_pTable = GetTable(dwVnum);
if (!c_pTable)
{
DWORD dwRaceFlag = 0;
return dwRaceFlag;
}
return c_pTable->dwRaceFlag;
}
DWORD CPythonNonPlayer::GetMonsterLevel(DWORD dwVnum)
{
const CPythonNonPlayer::TMobTable * c_pTable = GetTable(dwVnum);
if (!c_pTable)
{
DWORD level = 0;
return level;
}
return c_pTable->bLevel;
}
DWORD CPythonNonPlayer::GetMonsterDamage1(DWORD dwVnum)
{
const CPythonNonPlayer::TMobTable * c_pTable = GetTable(dwVnum);
if (!c_pTable)
{
DWORD range = 0;
return range;
}
return c_pTable->dwDamageRange[0];
}
DWORD CPythonNonPlayer::GetMonsterDamage2(DWORD dwVnum)
{
const CPythonNonPlayer::TMobTable * c_pTable = GetTable(dwVnum);
if (!c_pTable)
{
DWORD range = 0;
return range;
}
return c_pTable->dwDamageRange[1];
}
DWORD CPythonNonPlayer::GetMonsterExp(DWORD dwVnum)
{
const CPythonNonPlayer::TMobTable * c_pTable = GetTable(dwVnum);
if (!c_pTable)
{
DWORD dwExp = 0;
return dwExp;
}
return c_pTable->dwExp;
}
float CPythonNonPlayer::GetMonsterDamageMultiply(DWORD dwVnum)
{
const CPythonNonPlayer::TMobTable * c_pTable = GetTable(dwVnum);
if (!c_pTable)
{
DWORD fDamMultiply = 0;
return fDamMultiply;
}
return c_pTable->fDamMultiply;
}
DWORD CPythonNonPlayer::GetMonsterST(DWORD dwVnum)
{
const CPythonNonPlayer::TMobTable * c_pTable = GetTable(dwVnum);
if (!c_pTable)
{
DWORD bStr = 0;
return bStr;
}
return c_pTable->bStr;
}
DWORD CPythonNonPlayer::GetMonsterDX(DWORD dwVnum)
{
const CPythonNonPlayer::TMobTable * c_pTable = GetTable(dwVnum);
if (!c_pTable)
{
DWORD bDex = 0;
return bDex;
}
return c_pTable->bDex;
}
bool CPythonNonPlayer::IsMonsterStone(DWORD dwVnum)
{
const CPythonNonPlayer::TMobTable * c_pTable = GetTable(dwVnum);
if (!c_pTable)
{
DWORD bType = 0;
return false;
}
return c_pTable->bType == 2;
}
#endif
PythonNonPlayerModule.cpp
C:
#ifdef ENABLE_TARGET_INFORMATION_SYSTEM
PyObject * nonplayerGetMonsterMaxHP(PyObject * poSelf, PyObject * poArgs)
{
int race;
if (!PyTuple_GetInteger(poArgs, 0, &race))
return Py_BuildException();
CPythonNonPlayer& rkNonPlayer = CPythonNonPlayer::Instance();
return Py_BuildValue("i", rkNonPlayer.GetMonsterMaxHP(race));
}
PyObject * nonplayerGetRaceNumByVID(PyObject * poSelf, PyObject * poArgs)
{
int iVirtualID;
if (!PyTuple_GetInteger(poArgs, 0, &iVirtualID))
return Py_BuildException();
CInstanceBase * pInstance = CPythonCharacterManager::Instance().GetInstancePtr(iVirtualID);
if (!pInstance)
return Py_BuildValue("i", -1);
const CPythonNonPlayer::TMobTable * pMobTable = CPythonNonPlayer::Instance().GetTable(pInstance->GetVirtualNumber());
if (!pMobTable)
return Py_BuildValue("i", -1);
return Py_BuildValue("i", pMobTable->dwVnum);
}
PyObject * nonplayerGetMonsterRaceFlag(PyObject * poSelf, PyObject * poArgs)
{
int race;
if (!PyTuple_GetInteger(poArgs, 0, &race))
return Py_BuildException();
CPythonNonPlayer& rkNonPlayer = CPythonNonPlayer::Instance();
return Py_BuildValue("i", rkNonPlayer.GetMonsterRaceFlag(race));
}
PyObject * nonplayerGetMonsterLevel(PyObject * poSelf, PyObject * poArgs)
{
int race;
if (!PyTuple_GetInteger(poArgs, 0, &race))
return Py_BuildException();
CPythonNonPlayer& rkNonPlayer = CPythonNonPlayer::Instance();
return Py_BuildValue("i", rkNonPlayer.GetMonsterLevel(race));
}
PyObject * nonplayerGetMonsterDamage(PyObject * poSelf, PyObject * poArgs)
{
int race;
if (!PyTuple_GetInteger(poArgs, 0, &race))
return Py_BuildException();
CPythonNonPlayer& rkNonPlayer = CPythonNonPlayer::Instance();
DWORD dmg1 = rkNonPlayer.GetMonsterDamage1(race);
DWORD dmg2 = rkNonPlayer.GetMonsterDamage2(race);
return Py_BuildValue("ii", dmg1, dmg2);
}
PyObject * nonplayerGetMonsterExp(PyObject * poSelf, PyObject * poArgs)
{
int race;
if (!PyTuple_GetInteger(poArgs, 0, &race))
return Py_BuildException();
CPythonNonPlayer& rkNonPlayer = CPythonNonPlayer::Instance();
return Py_BuildValue("i", rkNonPlayer.GetMonsterExp(race));
}
PyObject * nonplayerGetMonsterDamageMultiply(PyObject * poSelf, PyObject * poArgs)
{
int race;
if (!PyTuple_GetInteger(poArgs, 0, &race))
return Py_BuildException();
CPythonNonPlayer& rkNonPlayer = CPythonNonPlayer::Instance();
return Py_BuildValue("f", rkNonPlayer.GetMonsterDamageMultiply(race));
}
PyObject * nonplayerGetMonsterST(PyObject * poSelf, PyObject * poArgs)
{
int race;
if (!PyTuple_GetInteger(poArgs, 0, &race))
return Py_BuildException();
CPythonNonPlayer& rkNonPlayer = CPythonNonPlayer::Instance();
return Py_BuildValue("i", rkNonPlayer.GetMonsterST(race));
}
PyObject * nonplayerGetMonsterDX(PyObject * poSelf, PyObject * poArgs)
{
int race;
if (!PyTuple_GetInteger(poArgs, 0, &race))
return Py_BuildException();
CPythonNonPlayer& rkNonPlayer = CPythonNonPlayer::Instance();
return Py_BuildValue("i", rkNonPlayer.GetMonsterDX(race));
}
PyObject * nonplayerIsMonsterStone(PyObject * poSelf, PyObject * poArgs)
{
int race;
if (!PyTuple_GetInteger(poArgs, 0, &race))
return Py_BuildException();
CPythonNonPlayer& rkNonPlayer = CPythonNonPlayer::Instance();
return Py_BuildValue("i", rkNonPlayer.IsMonsterStone(race) ? 1 : 0);
}
PyObject * nonplayerGetMobRegenCycle(PyObject * poSelf, PyObject * poArgs)
{
int race;
if (!PyTuple_GetInteger(poArgs, 0, &race))
return Py_BuildException();
CPythonNonPlayer& rkNonPlayer = CPythonNonPlayer::Instance();
return Py_BuildValue("i", rkNonPlayer.GetMobRegenCycle(race));
}
PyObject * nonplayerGetMobRegenPercent(PyObject * poSelf, PyObject * poArgs)
{
int race;
if (!PyTuple_GetInteger(poArgs, 0, &race))
return Py_BuildException();
CPythonNonPlayer& rkNonPlayer = CPythonNonPlayer::Instance();
return Py_BuildValue("i", rkNonPlayer.GetMobRegenPercent(race));
}
PyObject * nonplayerGetMobGoldMin(PyObject * poSelf, PyObject * poArgs)
{
int race;
if (!PyTuple_GetInteger(poArgs, 0, &race))
return Py_BuildException();
CPythonNonPlayer& rkNonPlayer = CPythonNonPlayer::Instance();
return Py_BuildValue("i", rkNonPlayer.GetMobGoldMin(race));
}
PyObject * nonplayerGetMobGoldMax(PyObject * poSelf, PyObject * poArgs)
{
int race;
if (!PyTuple_GetInteger(poArgs, 0, &race))
return Py_BuildException();
CPythonNonPlayer& rkNonPlayer = CPythonNonPlayer::Instance();
return Py_BuildValue("i", rkNonPlayer.GetMobGoldMax(race));
}
PyObject * nonplayerGetMobResist(PyObject * poSelf, PyObject * poArgs)
{
int race;
if (!PyTuple_GetInteger(poArgs, 0, &race))
return Py_BuildException();
BYTE resistNum;
if (!PyTuple_GetInteger(poArgs, 1, &resistNum))
return Py_BuildException();
CPythonNonPlayer& rkNonPlayer = CPythonNonPlayer::Instance();
return Py_BuildValue("i", rkNonPlayer.GetMobResist(race, resistNum));
}
#endif
/* ----------------------------------- ----------------------------------- */
#ifdef ENABLE_TARGET_INFORMATION_SYSTEM
// TARGET_INFO
{ "GetRaceNumByVID", nonplayerGetRaceNumByVID, METH_VARARGS },
{ "GetMonsterMaxHP", nonplayerGetMonsterMaxHP, METH_VARARGS },
{ "GetMonsterRaceFlag", nonplayerGetMonsterRaceFlag, METH_VARARGS },
{ "GetMonsterLevel", nonplayerGetMonsterLevel, METH_VARARGS },
{ "GetMonsterDamage", nonplayerGetMonsterDamage, METH_VARARGS },
{ "GetMonsterExp", nonplayerGetMonsterExp, METH_VARARGS },
{ "GetMonsterDamageMultiply", nonplayerGetMonsterDamageMultiply, METH_VARARGS },
{ "GetMonsterST", nonplayerGetMonsterST, METH_VARARGS },
{ "GetMonsterDX", nonplayerGetMonsterDX, METH_VARARGS },
{ "IsMonsterStone", nonplayerIsMonsterStone, METH_VARARGS },
{"GetMobRegenCycle", nonplayerGetMobRegenCycle, METH_VARARGS},
{"GetMobRegenPercent", nonplayerGetMobRegenPercent, METH_VARARGS},
{"GetMobGoldMin", nonplayerGetMobGoldMin, METH_VARARGS},
{"GetMobGoldMax", nonplayerGetMobGoldMax, METH_VARARGS},
{"GetResist", nonplayerGetMobResist, METH_VARARGS},
#endif
" ? " Tuşuna basınca açılacak yer;
uitarget.py
Python:
def __LoadInformation_Default(self, race):
self.AppendSeperator()
self.AppendTextLine(localeInfo.TARGET_INFO_MAX_HP % localeInfo.NumberToString(nonplayer.GetMonsterMaxHP(race)))
# calc att damage
monsterLevel = nonplayer.GetMonsterLevel(race)
fHitRate = self.__LoadInformation_Default_GetHitRate(race)
iDamMin, iDamMax = nonplayer.GetMonsterDamage(race)
iDamMin = int((iDamMin + nonplayer.GetMonsterST(race)) * 2 * fHitRate) + monsterLevel * 2
iDamMax = int((iDamMax + nonplayer.GetMonsterST(race)) * 2 * fHitRate) + monsterLevel * 2
iDef = player.GetStatus(player.DEF_GRADE) * (100 + player.GetStatus(player.DEF_BONUS)) / 100
fDamMulti = nonplayer.GetMonsterDamageMultiply(race)
iDamMin = int(max(0, iDamMin - iDef) * fDamMulti)
iDamMax = int(max(0, iDamMax - iDef) * fDamMulti)
if iDamMin < 1:
iDamMin = 1
if iDamMax < 5:
iDamMax = 5
self.AppendTextLine(localeInfo.TARGET_INFO_DAMAGE % (str(iDamMin), str(iDamMax)))
idx = min(len(self.EXP_BASE_LVDELTA) - 1, max(0, (monsterLevel + 15) - player.GetStatus(player.LEVEL)))
iExp = nonplayer.GetMonsterExp(race) * self.EXP_BASE_LVDELTA[idx] / 100
self.AppendTextLine(localeInfo.TARGET_INFO_EXP % localeInfo.NumberToString(iExp))
# self.AppendTextLine(localeInfo.TARGET_INFO_GOLD_MIN_MAX % (localeInfo.NumberToString(nonplayer.GetMobGoldMin(race)), localeInfo.NumberToString(nonplayer.GetMobGoldMax(race))))
self.AppendTextLine(localeInfo.TARGET_INFO_REGEN_INFO % (nonplayer.GetMobRegenPercent(race), nonplayer.GetMobRegenCycle(race)))
Source :
Linkleri görebilmek için
giriş yap veya kayıt ol.
En son bir moderatör tarafından düzenlenmiş: